見積書(複合帳票)
見積書の表紙+見積書本体の2枚構成の帳票のサンプルです。
ポイント
- 表紙に“header”, 本体に“body”という名前をつけることで,フィールドの名前空間を分離しています。
- 名前空間を挿入した分,context要素の階層を1段深くする必要があります。
- 日付の値として環境変数NOWを指定することで,現在日付を取得しています。
- 数値項目の値を数値とし,style要素により数値の書式を指定しています。
- style要素では,ワイルドカードを使うことで,復数フィールドに対して一括してstyleの指定を行っています。
Python
#!/usr/bin/env python
# coding: utf-8
import sys
from field import reports
param = {
# テンプレート
"template": [
{"header": "./hyousi.pdf"},
{"body": "./mitumori.pdf"}
],
# フィールド値
"context": {
"header": {
"date": "${NOW}",
"number": "10R0001",
"to": "△△△惣菜株式会社",
"title": "肉じゃがの材料",
"delivery_date": "2011-03-01",
"delivery_place": "貴社指定場所",
"payment_terms": "銀行振込",
"expiration_date": "発行から3ヶ月以内",
"total": 840
},
"body": {
"date": "${NOW}",
"number": "10R0001",
"to": "△△△惣菜株式会社",
"title": "肉じゃがの材料",
"delivery_date": "2011-03-01",
"delivery_place": "貴社指定場所",
"payment_terms": "銀行振込",
"expiration_date": "発行から3ヶ月以内",
"stamp1": {"icon": "./stamp.png"},
"table": [
["1", "N001", "牛肉(切り落とし)", "200g", 250, 500],
["2", "Y001", "じゃがいも(乱切り)", "3個", 30, 90],
["3", "Y002", "にんじん(乱切り)", "1本", 40, 40],
["4", "Y003", "たまねぎ(くし切り)", "1個", 50, 50],
["5", "Y004", "しらたき", "1袋", 80, 80],
["6", "Y005", "いんげん", "1袋", 40, 40]
],
"sub_total": 800,
"tax": 40,
"total": 840
}
},
# スタイル指定
"style": [
{"*.date": {"datetime": "GGE年M月D日"}},
{"*.delivery_date": {"datetime": "GGE年M月D日"}},
{"*.total": {"format": "###,###円"}},
{"*.sub_total": {"format": "###,###円"}},
{"*.tax": {"format": "###,###円"}},
{"body.table.*.[4:6]": {"format": "###,###円"}}
]
}
if __name__ == "__main__":
if len(sys.argv) == 2:
reports.set_log_level(3)
reports.render(param, sys.argv[1])
else:
print("usage: %s " % (sys.argv[0], ))
Ruby
#!/usr/bin/env ruby
# coding: utf-8
require 'rubygems'
require 'field/reports'
$param = {
# テンプレート
"template" => [
{"header" => "./hyousi.pdf"},
{"body" => "./mitumori.pdf"}
],
# フィールド値
"context" => {
"header" => {
"date" => "${NOW}",
"number" => "10R0001",
"to" => "△△△惣菜株式会社",
"title" => "肉じゃがの材料",
"delivery_date" => "2011-03-01",
"delivery_place" => "貴社指定場所",
"payment_terms" => "銀行振込",
"expiration_date" => "発行から3ヶ月以内",
"total" => 840
},
"body" => {
"date" => "${NOW}",
"number" => "10R0001",
"to" => "△△△惣菜株式会社",
"title" => "肉じゃがの材料",
"delivery_date" => "2011-03-01",
"delivery_place" => "貴社指定場所",
"payment_terms" => "銀行振込",
"expiration_date" => "発行から3ヶ月以内",
"stamp1" => {"icon" => "./stamp.png"},
"table" => [
["1", "N001", "牛肉(切り落とし)", "200g", 250, 500],
["2", "Y001", "じゃがいも(乱切り)", "3個", 30, 90],
["3", "Y002", "にんじん(乱切り)", "1本", 40, 40],
["4", "Y003", "たまねぎ(くし切り)", "1個", 50, 50],
["5", "Y004", "しらたき", "1袋", 80, 80],
["6", "Y005", "いんげん", "1袋", 40, 40]
],
"sub_total" => 800,
"tax" => 40,
"total" => 840
}
},
# スタイル指定
"style" => [
{"*.date" => {"datetime" => "GGE年M月D日"}},
{"*.delivery_date" => {"datetime" => "GGE年M月D日"}},
{"*.total" => {"format" => "###,###円"}},
{"*.sub_total" => {"format" => "###,###円"}},
{"*.tax" => {"format" => "###,###円"}},
{"body.table.*.[4:6]" => {"format" => "###,###円"}}
]
}
if ARGV.length == 1 then
Field::Reports.set_log_level(3)
Field::Reports.render($param, ARGV[0])
else
p "usage: %s " % $0
end
Perl
#!/usr/bin/env perl
# coding: utf-8
use utf8;
use Field::Reports;
my $param = {
# テンプレート
"template" => [
{"header" => "./hyousi.pdf"},
{"body" => "./mitumori.pdf"}
],
# フィールド値
"context" => {
"header" => {
"date" => "\${NOW}",
"number" => "10R0001",
"to" => "△△△惣菜株式会社",
"title" => "肉じゃがの材料",
"delivery_date" => "2011-03-01",
"delivery_place" => "貴社指定場所",
"payment_terms" => "銀行振込",
"expiration_date" => "発行から3ヶ月以内",
"total" => 840
},
"body" => {
"date" => "\${NOW}",
"number" => "10R0001",
"to" => "△△△惣菜株式会社",
"title" => "肉じゃがの材料",
"delivery_date" => "2011-03-01",
"delivery_place" => "貴社指定場所",
"payment_terms" => "銀行振込",
"expiration_date" => "発行から3ヶ月以内",
"stamp1" => {"icon" => "./stamp.png"},
"table" => [
["1", "N001", "牛肉(切り落とし)", "200g", 250, 500],
["2", "Y001", "じゃがいも(乱切り)", "3個", 30, 90],
["3", "Y002", "にんじん(乱切り)", "1本", 40, 40],
["4", "Y003", "たまねぎ(くし切り)", "1個", 50, 50],
["5", "Y004", "しらたき", "1袋", 80, 80],
["6", "Y005", "いんげん", "1袋", 40, 40]
],
"sub_total" => 800,
"tax" => 40,
"total" => 840
}
},
# スタイル指定
"style" => [
{"*.date" => {"datetime" => "GGE年M月D日"}},
{"*.delivery_date" => {"datetime" => "GGE年M月D日"}},
{"*.total" => {"format" => "###,###円"}},
{"*.sub_total" => {"format" => "###,###円"}},
{"*.tax" => {"format" => "###,###円"}},
{"body.table.*.[4:6]" => {"format" => "###,###円"}}
]
};
if (@ARGV == 1) {
Field::Reports::set_log_level(3);
Field::Reports::render($param, $ARGV[0]);
} else {
die "usage: $0 \n";
}
PHP
<?php
$param = array(
# テンプレート
"template" => array(
array("header" => "./hyousi.pdf"),
array("body" => "./mitumori.pdf")
),
# フィールド値
"context" => array(
"header" => array(
"date" => "\${NOW}",
"number" => "10R0001",
"to" => "△△△惣菜株式会社",
"title" => "肉じゃがの材料",
"delivery_date" => "2011-03-01",
"delivery_place" => "貴社指定場所",
"payment_terms" => "銀行振込",
"expiration_date" => "発行から3ヶ月以内",
"total" => 840
),
"body" => array(
"date" => "\${NOW}",
"number" => "10R0001",
"to" => "△△△惣菜株式会社",
"title" => "肉じゃがの材料",
"delivery_date" => "2011-03-01",
"delivery_place" => "貴社指定場所",
"payment_terms" => "銀行振込",
"expiration_date" => "発行から3ヶ月以内",
"stamp1" => array("icon" => "./stamp.png"),
"table" => array(
array("1", "N001", "牛肉(切り落とし)", "200g", 250, 500),
array("2", "Y001", "じゃがいも(乱切り)", "3個", 30, 90),
array("3", "Y002", "にんじん(乱切り)", "1本", 40, 40),
array("4", "Y003", "たまねぎ(くし切り)", "1個", 50, 50),
array("5", "Y004", "しらたき", "1袋", 80, 80),
array("6", "Y005", "いんげん", "1袋", 40, 40)
),
"sub_total" => 800,
"tax" => 40,
"total" => 840
)
),
# スタイル指定
"style" => array(
array("*.date" => array("datetime" => "GGE年M月D日")),
array("*.delivery_date" => array("datetime" => "GGE年M月D日")),
array("*.total" => array("format" => "###,###円")),
array("*.sub_total" => array("format" => "###,###円")),
array("*.tax" => array("format" => "###,###円")),
array("body.table.*.[4:6]" => array("format" => "###,###円"))
)
);
fr_set_log_level(3);
fr_render($param, $argv[1]);
?>
実行結果
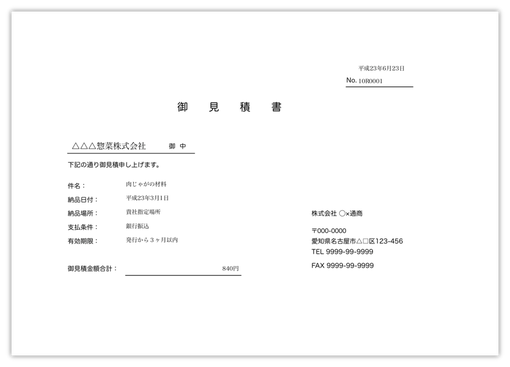
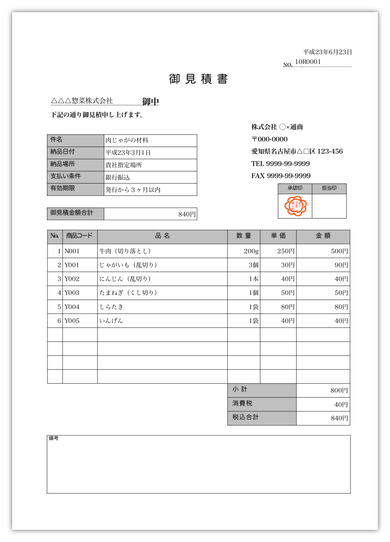